Child to Parent Event communication in LWC : jayakrishnasfdc
by: jayakrishnasfdc
blow post content copied from Jayakrishna Ganjikunta
click here to view original post
To Create an event use [CustomEvent()
constructor]and also Dispatch the Event use [EventTarget.dispatchEvent() method]
- Handle an Event : There are two ways to listen to an event
- Declarative via html markup : We need to add “on” prefix in the event name in Parent Component during calling of Child Component for Declaratively event listener.
- JavaScript using addEventListener method : We can explicitly attach a listener by using the addEventListner method in the parent component like below :this.template.addEventListener(‘eventName’, this.handleNotification.bind(this));
How to do with Declarative via html markup
- Create one child component component from where we will raise a event
- Create child html file to get value from user.
childComp.html - Now update Child Comp javaScript file to raise a CustomEvent with text value
childComp.js
import { LightningElement } from ‘lwc’;
export default class ChildComp extends LightningElement {
handleChange(event) {
event.preventDefault();
const name = event.target.value;
const selectEvent = new CustomEvent(‘mycustomevent’, {
detail: name
});
this.dispatchEvent(selectEvent);
}
}
- Create one Parent component where we will handle the event
- Now create parent component. We need to add prefix as “on” before the custom event name and in parent component we need to invoke the event listener as handleCustomEvent using onmycustomevent attribute.
ParentComponent.html - Now update parent component javaScript file and add handleCustomEvent method.
ParentComponent.js
import { LightningElement , track } from ‘lwc’;
export default class ParentComponent extends LightningElement {
@track msg;
handleCustomEvent(event) {
const textVal = event.detail;
this.msg = textVal;
}
JavaScript using addEventListener method | Attaching event Listener Programmatically
Update parent component JavaScript like below.
ParentComponent.js
import { LightningElement , track } from ‘lwc’;
export default class ParentComponent extends LightningElement {
@track msg;
constructor() {
super();
this.template.addEventListener(‘mycustomevent’, this.handleCustomEvent.bind(this));
}
handleCustomEvent(event) {
const textVal = event.detail;
this.msg = textVal;
}
}
Set the bubbles: true while raising the event like below in childComp.js
import { LightningElement } from ‘lwc’;
export default class ChildComp extends LightningElement {
handleChange(event) {
event.preventDefault();
const name = event.target.value;
const selectEvent = new CustomEvent(‘mycustomevent’, {
detail: name , bubbles: true
});
this.dispatchEvent(selectEvent);
}
}
Example:
childComponent.html
I am passing the input value to parent component where childComponent is called.
<template> <lightning-input onchange={handleChnage} type="number" name="input1" label="Enter a number" ></lightning-input> </template>
childComponent.js
We will get the value enteredas input on change event and passing the data to parent component by creating a custom event with data using detail and dispatching it.
import { LightningElement, api } from "lwc"; export default class ChildComponent extends LightningElement { @api progressValue; handleChnage(event) { this.progressValue = event.target.value; // Creates the event with the data. const selectedEvent = new CustomEvent("progressvaluechange", { detail: this.progressValue }); // Dispatches the event. this.dispatchEvent(selectedEvent); } }
childComponent.js-meta.xml
No changes required.
I am creating now Parent component where i am going to call the childComponent and handling the event.
parentComponent.html
We have a progress bar which gets the value from childComponent. We are handling the event in parentComponent and getting the data that have been dispatched with custom event.
<template> <lightning-card title="Getting Data From Child"> <p class="slds-p-horizontal_small"> <lightning-progress-bar value={progressValue} size="large" ></lightning-progress-bar> <c-child-lwc onprogressvaluechange={hanldeProgressValueChange} ></c-child-Component> </p> </lightning-card> </template>
parentComponent.js
We are setting the value received from child to progress bar indicator. event.detail gives the value comes with custom event.
import { LightningElement, track } from "lwc"; export default class ParentComponent extends LightningElement { @track progressValue = 0; hanldeProgressValueChange(event) { this.progressValue = event.detail; } }
parentComponent.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="parentComponent"> <apiVersion>46.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__RecordPage</target> <target>lightning__AppPage</target> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>
December 05, 2020 at 04:36PM
Click here for more details...
=============================
The original post is available in Jayakrishna Ganjikunta by jayakrishnasfdc
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
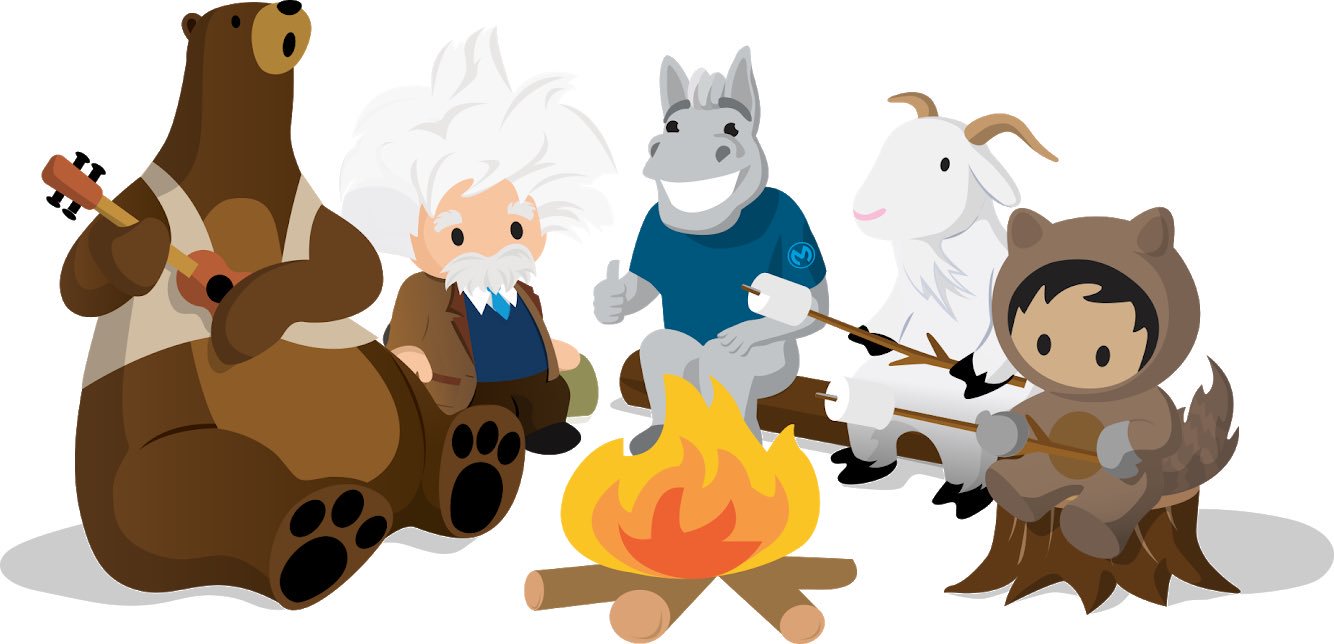
Post a Comment